FastLED 16x16 LED matrix generator
Generator and example code
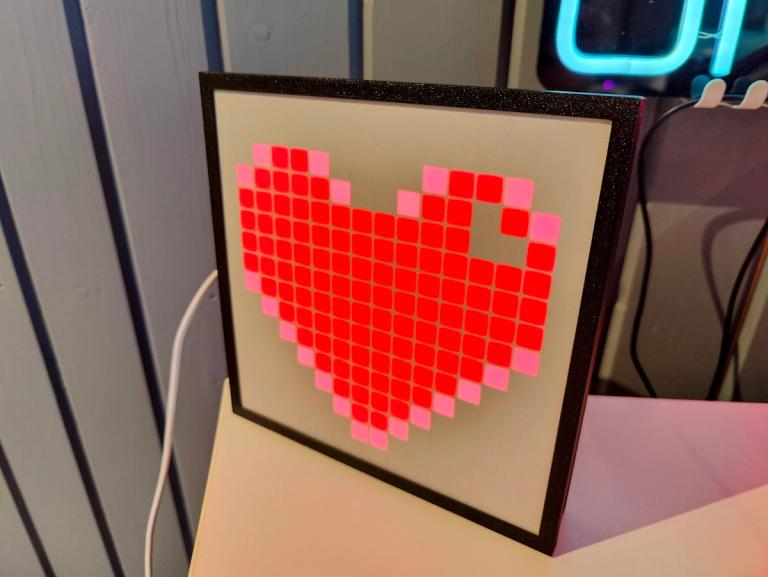
FastLED 16x16 matrix generator is a simple generator for creating simple pixel art for 16x16 matrix LED panels in combination with Arduino compatible board.
You will find the generator itself at https://fastledmatrixgenerator.netlify.app/ I assume that you are already familiar with Arduino and basic programming as well as connecting the matrix.
Explanation of the generator:
In the generator you can choose a color and which square (LED) should have that color. That way you can draw your own pixel art.
The generator then creates an array of objects that can be pasted into the .INO file. The array contains which LED and the RGB color code for each LED in the matrix.
Arduino code:
#include "FastLED.h"
#define NUM_LEDS 256
#define DATA_PIN 5
CRGB leds[NUM_LEDS];
struct Color {
uint8_t r; // Red
uint8_t g; // Green
uint8_t b; // Blue
};
struct Data {
int num; // Led
Color color; // Color
};
Data data[] = {}; // Paste generated code here
const int dataSize = sizeof(data) / sizeof(data[0]);
void setup() {
delay(2000);
FastLED.addLeds<WS2812B, DATA_PIN, RGB>(leds, NUM_LEDS);
FastLED.setBrightness(90); //Number 0-255
FastLED.clear();
}
void loop() {
delay(1000);
for (int i = 0; i < dataSize; i++) {
leds[data[i].num].r = data[i].color.g;
leds[data[i].num].g = data[i].color.r;
leds[data[i].num].b = data[i].color.b;
}
FastLED.show();
}
A little more explanation
... of the code and the generator. This code may not be right for you. I recommend that you familiarize yourself with FastLED and your matrix. For my matrix, LED no. 1 is at the bottom right and goes in a zigzag pattern in the columns.
Another small change is that I had to swap the red and green colors in the code. So if you get the wrong colors, you can correct this in the code.